Understanding Quicksort in Kotlin
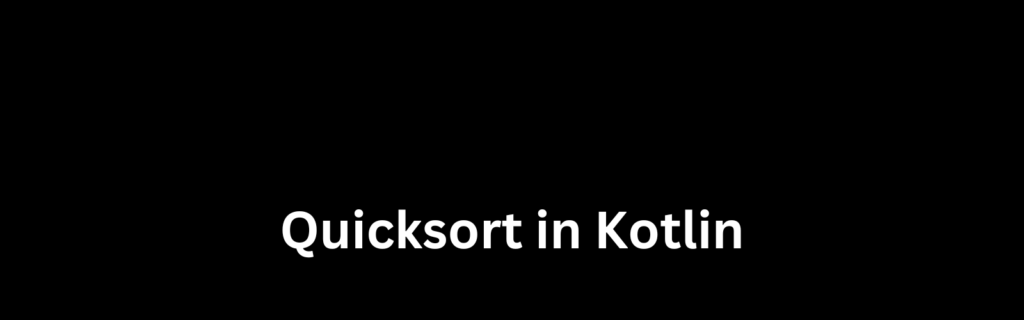
Quicksort is a widely-used sorting algorithm known for its efficiency and simplicity. In this article, we’ll delve into the inner workings of Quicksort and implement it in Kotlin. Introduction to Quicksort Quicksort is a comparison sort algorithm that follows the divide-and-conquer paradigm. It was developed by Tony Hoare in 1960 and is widely used due […]
Bubble Sort in Kotlin: A Comprehensive Guide
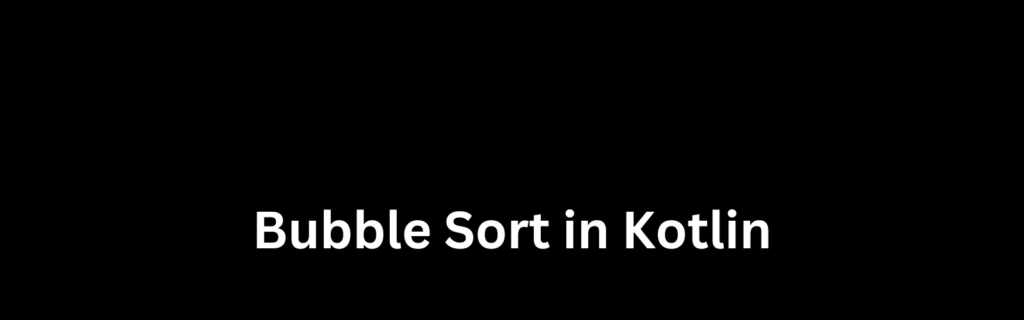
Introduction Sorting is a fundamental operation in computer science, and various algorithms have been developed to efficiently organize data. One such elementary sorting algorithm is Bubble Sort. In this article, we will explore Bubble Sort and implement it using the Kotlin programming language. Understanding Bubble Sort Bubble Sort is a simple comparison-based sorting algorithm that […]
Converting Kotlin Array to Varargs
Introduction Kotlin, a modern programming language for the Java Virtual Machine (JVM), offers concise syntax and powerful features. When working with arrays in Kotlin, you may encounter situations where you need to convert an array into varargs (variable-length argument lists). This conversion is essential for passing array elements as individual arguments to functions that accept […]
Understanding ArrayDeque in Kotlin
Introduction In Kotlin, the ArrayDeque class is a part of the standard library and represents a resizable, double-ended queue. This data structure allows elements to be added or removed from both ends efficiently. The ArrayDeque is implemented using a resizable array and provides constant-time amortized complexity for adding or removing elements from both ends. Initializing […]
How to Work With List Casts in Kotlin
Kotlin is a versatile and expressive programming language that provides numerous features to help developers write clean and concise code. One common operation in Kotlin is working with lists, and sometimes, you may need to cast elements within a list to different types. This article will guide you through the process of working with list […]
Getting the Difference Between Two Lists in Kotlin
Kotlin is a modern, statically-typed programming language that has gained popularity for its concise and expressive syntax. When working with lists in Kotlin, you may encounter situations where you need to find the difference between two lists. This could be for various purposes, such as identifying elements that exist in one list but not in […]
Kotlin-Java Interoperability: Bridging the Gap
In the world of software development, programming languages often coexist within a project or ecosystem. Kotlin, a statically typed programming language developed by JetBrains, has gained significant popularity for its modern features, concise syntax, and robust safety mechanisms. However, it’s common for Kotlin projects to need to interact with existing Java codebases or libraries. This […]
Exploring Try-with-resources in Kotlin
Exception handling and resource management are crucial aspects of any programming language. Kotlin, a modern and concise programming language for the JVM and Android development, offers a convenient and concise way to handle resources using the try-with-resources construct. This construct allows developers to elegantly manage resources that need to be closed or released after their […]
Modifying Kotlin Lists In-Place
In Kotlin, lists are a fundamental data structure used to store collections of elements. These lists are immutable by default, meaning their contents cannot be changed once they are created. However, there are scenarios where you might need to modify the elements of a list directly, without creating a new list. Kotlin provides several techniques […]
Difference Between fold and reduce in Kotlin
In Kotlin, functional programming constructs such as fold and reduce provide powerful tools for working with collections. Both functions allow you to accumulate values from a collection, but they differ in subtle yet significant ways. In this article, we’ll delve into the differences between fold and reduce, explore their use cases, and provide relevant code […]